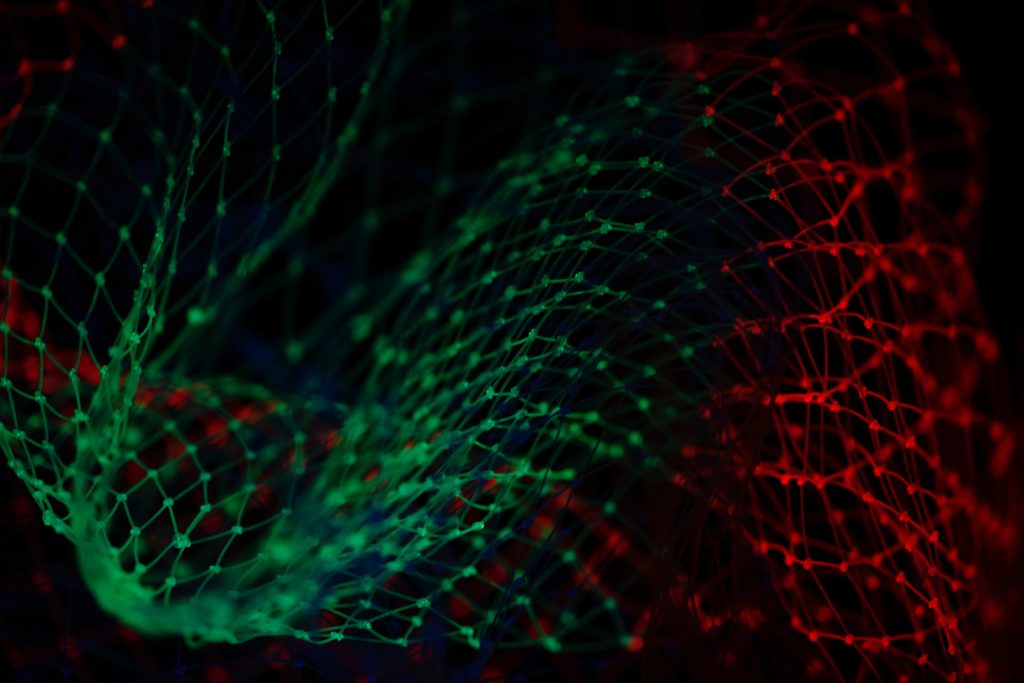
Introduction to a smart contract on blockchain
Table of Contents
A smart contract is a computer protocol intended to facilitate, verify, or enforce the negotiation or performance of a contract. Since the launch of Ethereum, we deal with a smart contract on blockchain which is a kind of computer programm that is stored on a blockchain and is used to automate the execution of an agreement. Let’s see how it works!
Smart Contract on blockchain – needed knowledge
Before we start creating example of a smart contract on blockchain I would like to introduction some terminology that you should known. What technologies and tools we need to create and deploy a Smart Contract to the Ethereum network. In this article I will show you how source code looks like, how to compile with Solidity compiler. Also how to create private network for testing.
You should know basic knowledge about:
- Javascript
- Linux and CLI
- Solidity
- Ethereum network
Tools we need for a smart contract on blockchain
- NodeJS
- Solidity compiler (solcjs)
- Ethereum client: geth
To write a smart contract on blockchain we need editor. I prefer Web IDE called Remix. Remix gives us possibility to write, compile and deploy our Contract. It also support JavaScript Virtual Machine – so we don’t really need deploy our code to the network. It is good for testing, but does not reflect a full network (e.g. with time of block mining). In this article we will use private network. Remix have option to set Web3 Provider, so then we can use our network.
Ethereum have multiple clients. Mentioned above geth but also Parity or cpp_ethereum. Parity is good for general group of users because it have Web UI, where we can find your wallet, transactions list, transactions queues even console.
Private Network
To create private Ethereum network and blockchain we need Ethereum Client (called nodes). Ethereum network is Peer to Peer network. In minimal scenario we should have only one node. It can be installed on our private computer. When we have multiple developers in a project, every developer should install the client. Then they connect to one P2P network. In this scenario it would be good to have master client, for example installed on server. Also it would be good to have one global miner, who will be confirm (mining) transactions.
Configuration of private network
Every network have configuration of genesis block. Genesis block is the first block in blockchain, and it does not have hash of previous block. For Ethereum network there must exists genesis.json file.
{ "config": { "chainId": 2456, "homesteadBlock": 0, "eip155Block": 0, "eip158Block": 0 }, "difficulty": "1", "gasLimit": "2100000", "alloc": { "0a824020dca880540874976c451bf7c372bd9a22": { "balance": "1000000000000000000" } } }
In this file there is an option to define our networkId (chainId), information about some improvement (eips) with block number when change will be online on the network. It is mainly for main network. What else? Definition of starting difficulty and gas limit. You can also predefine addresses with starting balances.
Starting the network
As mentioned above we need some client. We will use geth, because we do not need the UI. Only CLI. Geth can be downloaded from official website: Geth & Tools.
To start network we should generate some node.key. It will be used to generate enode url. That url is used to connection P2P between nodes.
bootnode --genkey node.key
Next step is to generate some new Ethereum account in our client.
./geth --datadir=./ethereum account new
Of course our new generated account we can define in genesis.json.
Starting the client without initialize genesis will initialize main-net genesis block. To do define custom genesis block we should run:
./geth --datadir=./ethereum --networkid 2456 --nodekey node.key init genesis.json
As you can see, we using the same chainId number in networkid parameter, node.key file and genesis.json. After that we can start our client without init genesis.json fragment but we need to enable mine option: –mine –minerthreads 1 –etherbase <yours ethereum address>. And client will have P2P address based on our node.key. It will be looks like that:
self=enode://979d6e5410410581a0930dbd2bfdd31e1e4fb7425f724721fe46ca482b7462a3179b79dfc816bb6ea3613925841d283645f3e13813a5c1b1e24304e672f7b46e@[::]:30303
Smart Contract on blockchain
Now, when we have our client up and running we can try create Hello world contract. To do such thing we need to have source code of contract and as mentioned above some compiler. Compiler will compile code to the byte-code and also it will generate Application Binary Interface (ABI). ABI will be needed to do any operation on the deployed Smart Contract on blockchain. Example source code looks like this:
pragma solidity ^0.4.17; contract Hello { string _text; function Hello(string text) public { _text = text; } function showText() public constant returns(string) { return _text; } }
Compile – How-to
To compile the source code we need install compiler.
npm install -g solc
And generate binary code and ABI code.
solcjs Hello.sol --bin solcjs Hello.sol --abi
Deployment script
Next step in deployment is to create javascript file with deployment script. In this script we must set ABI and binary data ( place).
var text = "Hello World"; var helloContract = web3.eth.contract([{ "constant": true, "inputs": [], "name": "showText", "outputs": [{"name": "", "type": "string"}], "payable": false, "stateMutability": "view", "type": "function" }, { "inputs": [{"name": "text", "type": "string"}], "payable": false, "stateMutability": "nonpayable", "type": "constructor" }]); var hello = helloContract.new( text, { from: web3.eth.accounts[0], data: '<BIN DATA>', gas: '2100000' }, function (e, contract) { console.log(e, contract); if (typeof contract.address !== 'undefined') { console.log('Contract mined! address: ' + contract.address + ' transactionHash: ' + contract.transactionHash); } });
We define text to Hello World. This variable will be executed as parameter of constructor. And showText() function should return this text.
Then this script will be directly loaded to geth console. When geth is running we can attach with command: geth attach. Then we just execute:
loadScript("deploy.js")
After couple seconds we will see information about submitted contract creation and if contract will be mined then some Ethereum address will be generated.
Contract mined! address: 0x1fbc3e8b42f9b9ea7ca3df8057f47c2d58226e00 transactionHash: 0x347f83c32bc41f862009470e5f35dfea3e1a535d1cb20c5622c8b60ff0377e07
After deployment
When we successfully deployed our first contract, we can use ABI to load contract and execute some action. To do this we need to again attach to the client console and just run:
var hello = eth.contract([{"constant":true,"inputs":[],"name":"showText","outputs":[{"name":"","type":"string"}],"payable":false,"stateMutability":"view","type":"function"},{"inputs":[{"name":"text","type":"string"}],"payable":false,"stateMutability":"nonpayable","type":"constructor"}]).at("0x1fbc3e8b42f9b9ea7ca3df8057f47c2d58226e00")
After this we can execute our showText() function.
hello.showText() "Hello World”
This may interest you:
Concise Software among the best blockchain developers in Poland!
Blockchain in healthcare – here are 5 essential use cases
10 Use Cases of Blockchain in Banking
How are we all going to use blockchains in the near future?
Blockchain – a starter guide to the world of technology
Cryptocurrencies and “mining” – how does the digging process work?
Everything you need to know about Ethereum
Open banking – a new dimension of financial services
Smart contracts – what are they and how to create them? [GUIDE]
P2P payments – what are peer-to-peer payment apps used for?
What is an Initial Exchange Offering (IEO) and how to participate in it?
IEO vs STO – key differences you should know
What is blockchain development? A complete guide!