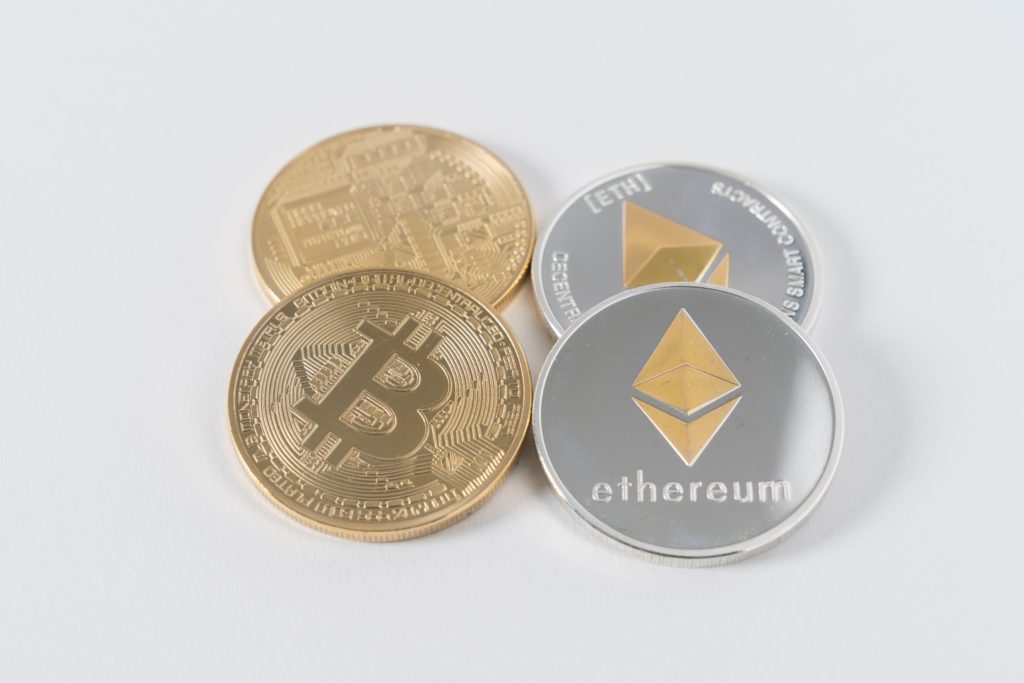
Ethereum Token – ERC20
Table of Contents
The most popular engine, where we can create tokens is Ethereum. It is not only a cryptocurrency but also a development platform. With Ethereum, every user can use blockchain technology. Everyone can create DApps (Decentralized Application) with the usage of Smart Contracts. One possibility of use Smart Contract is creating an Ethereum Token. Nowadays Ethereum community created a standard implementation for Ethereum Tokens – called ERC20.
In this article, we will tell you a bit about Ethereum Tokens based on Smart Contracts. For developing tokens is good to know basics about Solidity – which is a programming language of contracts – also with a tool like Remix. It is also good to know basics about Ethereum Network itself.
What is Ethereum Token?
Tokens like cryptocurrencies represent some virtual value. Ethereum Tokens can have different purposes. Most of them are available on cryptocurrencies market, so they have value in FIAT currency. Some of the tokens can be used as the payment medium (like US Dollar). Some of them can be used for ICO (Initial Coin Offering). Others may serve as a reflection of the ownership of a certain percentage of ownership. Also, it is important to notice, that Token is managed by code, not by person. With this is possible for developers to create functionality to make inflation with creation more token in time.
Implementation of an Ethereum Token
At the start of Ethereum, tokens don’t have formal standardization. That means every implementation can have different behavior. Functionality then can’t be support by 3rd party tools or cryptocurrency markets. Ethereum community worked out a standard interface implementation for tokens. This implementation is called ERC20. The ERC20 standard defines based functionality and implementation. It also gives functionality to make the transfer by 3rd persons.
The ERC20 standard defines functionality like:
- name – return the name of the token: eg. MyToken
function name() constant returns (string name) - symbol – return short tag of the token: np. MTO
function symbol() constant returns (string symbol) - decimals – decimal points for a token. Used mostly for representation. It’s like Wei to Ether.
function decimals() constant returns (uint8 decimals) - totalSupply – total amount of created tokens
function totalSupply() constant returns (uint256 totalSupply) - balanceOf – balance of tokens on given address
function balanceOf(address _owner) constant returns (uint256 balance) - transfer – function for transfer tokens to other accounts. The function must return event
Transfer when the transfer was successful. Must throw an error when we don’t have the necessary amount of funds.
function transfer(address _to, uint256 _value) returns (bool success) - transferFrom – function for transfer token from one account to other by 3rd party.
function transferFrom(address _from, address _to, uint256 _value) returns (bool success) - approve – give access to another party to execute transfer from our account to other
function approve(address _spender, uint256 _value) returns (bool success) - allowance – return amount what 3rd party can transfer to other by using transferFrom functionality.
function allowance(address _owner, address _spender) constant returns (uint256 remaining)
Events:
- Transfer – event executed when a transfer occurs.
- Approval – event executed when approve occurs.
It is important to notice, that implementation of ERC20 Tokens can be different in details. Can have more functionality (eg. freeze tokens on accounts or automatic give tokens for Ether transfer). Depending on the assumptions of the creators, they can support more security or put a lot of emphasis on saving the gas used by the contract.
Needed tools
Mentioned at the start of this article tool what we will use is Remix. The Remix is a Web IDE for developing Smart Contract. It also has functionality for compilation and deploying contract. If necessary we can debug transactions. Without configured private network or connection to Ethereum Test Networks, we can use Remix’s Javascript Virtual Machine.
Source code of ERC20 Token
In next part, there is a source code of ERC20 Token. This implementation is simple without additional functionalities. For our article, we will create CSToken – in short CST. The total amount of tokens we will define as 1000000. Out decimal points, we will define to 18.
We need definition of ERC20 interface:
./ERC20Interface.sol:
pragma solidity ^0.4.18; contract ERC20Interface { uint256 public totalSupply; function balanceOf(address _owner) public view returns (uint256 balance); function transfer(address _to, uint256 _value) public returns (bool success); function transferFrom(address _from, address _to, uint256 _value) public returns (bool success); function approve(address _spender, uint256 _value) public returns (bool success); function allowance(address _owner, address _spender) public view returns (uint256 remaining); event Transfer(address indexed _from, address indexed _to, uint256 _value); event Approval(address indexed _owner, address indexed _spender, uint256 _value); }
Next step is implementation of token: ./CSToken.sol:
pragma solidity ^0.4.18; import "./ERC20Interface.sol"; contract CSToken is ERC20Interface { uint256 constant private MAX_UINT256 = 2**256 - 1; mapping (address => uint256) public balances; mapping (address => mapping (address => uint256)) public allowed; /// Name of token string public name; /// Decimal points of our token. If we define totalSupply as 1 and decimal to 18 our total amount of token will /// be: 1000000000000000000 (1 * 10^18) uint8 public decimals; /// Three or four symbol of token string public symbol; /// Constructor of token. All defined tokens in _initialAmount will be bind to creator of contract. function CSToken(uint256 _initialAmount, string _tokenName, uint8 _decimalUnits, string _tokenSymbol) public { symbol = _tokenSymbol; name = _tokenName; decimals = _decimalUnits; totalSupply = _initialAmount * 10**uint256(decimals); balances[msg.sender] = totalSupply; } /// Check user balance function balanceOf(address _owner) public view returns (uint256 balance) { return balances[_owner]; } /// Transfer token from our wallet to other function transfer(address _to, uint256 _value) public returns (bool success) { require(balances[msg.sender] >= _value); balances[msg.sender] -= _value; balances[_to] += _value; Transfer(msg.sender, _to, _value); return true; } /// Transfer tokens from one account to other by 3rd party. function transferFrom(address _from, address _to, uint256 _value) public returns (bool success) { uint256 allowance = allowed[_from][msg.sender]; require(balances[_from] >= _value && allowance >= _value); balances[_to] += _value; balances[_from] -= _value; if (allowance < MAX_UINT256) { allowed[_from][msg.sender] -= _value; } Transfer(_from, _to, _value); return true; } /// Allow possiblity to transfer our tokens by 3rd party with maximum limit of _value. function approve(address _spender, uint256 _value) public returns (bool success) { allowed[msg.sender][_spender] = _value; Approval(msg.sender, _spender, _value); return true; } function allowance(address _owner, address _spender) public view returns (uint256 remaining) { return allowed[_owner][_spender]; } // Don’t accept ETH function () public { revert(); } }
Deployment of Ethereum Token
With using of Remix IDE we can edit and deploy our code. To create contract we will define starting parameters of a token as:
- The total amount of tokens: 1000000,
- Name of token: “CSToken”,
- Decimal points: 18 – its rather a standard approach,
- The short name of token: “CST”.
So given parameters for constructor will be: 1000000,”CSToken”,18,”CST”. After deploy we will get new contract address. Next possibility is to using our contract through Remix. Finished amount of tokens should be 100,000,000,000,000,000,000,000 as we defined 18 decimal points.
While we got our address we can use now 3rd party tools. So we use Mist for wallet management.
In Mist we can track tokens what we have on our account. We can transfer tokens to other account using transfer function. If we want to send 50 tokens to another person, we must provide a parameter to function as result of 50 * 1018. After transfer, our token should be tracked by Mist.
Summary
The token is next opportunity given by blockchain technology. Thanks to ERC20 standard 3rd party tools gave greatest possibilities to management or tracking. That standard gives the possibility to usage tokens directly on cryptocurrencies market. That mean tokens can have real market value. Also, having tokens can be a proof of a certain amount of resources – because a token can reflect, for example, shares.
You may like to read:
What are security tokens? Here’s everything you need to know
Types of tokens – what do you need to know? Concise Software Guide
Security Token Offering – is it good for you and your company?